源码:
#路飞骷髅
import turtle as t
#黄底帽子
t.pu()
t.goto(0,200)
t.circle(-130,-80)
t.pd()
t.colormode(255)
t.pensize(5)
t.color(242,232,184) #帽子黄底RGB
t.begin_fill()
t.pencolor(0,0,0)
t.circle(-130,160)
t.seth(180)
t.fd(255)
t.end_fill()
#红色线条
t.begin_fill()
t.color(221,65,43) #帽子红色带
t.pencolor(0,0,0)
t.seth(80)
t.circle(-130,19)
t.seth(0)
t.fd(225)
t.seth(-59)
t.circle(-130,19)
t.seth(180)
t.fd(255)
t.end_fill()
#帽檐
t.begin_fill()
t.color(242,232,184)
t.pencolor(0,0,0)
t.fd(60)
t.circle(12,180)
t.fd(375)
t.circle(12,180)
t.fd(255 + 60)
t.end_fill()
#脸部下半轮廓
t.pu()
t.setpos(0,-30)
t.seth(-180)
t.circle(-130,-75)
t.pd()
t.circle(-130,150)
#眼睛鼻子
t.pu()
t.color(33,24,24) #眼睛、鼻子RGB
t.setpos(-45,64)
t.seth(-180)
t.pd()
t.begin_fill()
t.circle(33)
t.pu()
t.setpos(45,64)
t.pd()
t.circle(33)
t.end_fill()
t.pu()
t.setpos(0,5)
t.pd()
t.begin_fill()
t.circle(8)
t.end_fill()
#下巴
t.pencolor(0,0,0)
t.pu()
t.setpos(0,0)
t.seth(0)
t.circle(-75,45)
t.pd()
t.circle(-75,270)
#牙齿
t.pu()
t.setpos(0,120)
t.seth(0)
t.circle(-105,136)
t.pd()
t.circle(-105,86)
t.pu()
t.seth(0)
t.goto(0,200)
t.circle(-130,150)
t.pd()
t.circle(-130,60)
t.pu() #牙齿三根竖线
t.setpos(-30,-27)
t.seth(260)
t.pd()
t.fd(52)
t.pu()
t.setpos(30,-27)
t.pd()
t.seth(-260)
t.fd(-52)
t.pu()
t.setpos(0,-30)
t.seth(-90)
t.pd()
t.fd(56)
#上排右侧小爪爪
#释放注释为:上排右侧小爪爪实心金方案
t.pu()
#t.color(255,215,0) #金色的RGB
t.pencolor(0,0,0)
t.setpos(110,145)
t.seth(45)
t.pd()
#t.begin_fill()
t.fd(40)
t.seth(135)
t.circle(-30,235)
t.seth(-20)
t.circle(-30,220)
t.seth(-135)
t.fd(40)
#t.end_fill()
#上排左侧小爪爪
t.pu()
t.pencolor(0,0,0)
t.setpos(-110,145)
t.seth(135)
t.pd()
t.fd(40)
t.seth(45)
t.circle(30,235)
t.seth(-160)
t.circle(30,220)
t.seth(-45)
t.fd(40)
#下排右侧小爪爪
t.pu()
t.setpos(70,-10)
t.seth(-45)
t.pd()
t.fd(70)
t.seth(45)
t.circle(-30,235)
t.seth(-70)
t.circle(-30,255)
t.seth(135)
t.fd(22)
#下排左侧小爪爪
t.pu()
t.setpos(-70,-10)
t.seth(-135)
t.pd()
t.fd(70)
t.seth(135)
t.circle(30,235)
t.seth(-110)
t.circle(30,255)
t.seth(45)
t.fd(22)
t.done()
效果图:
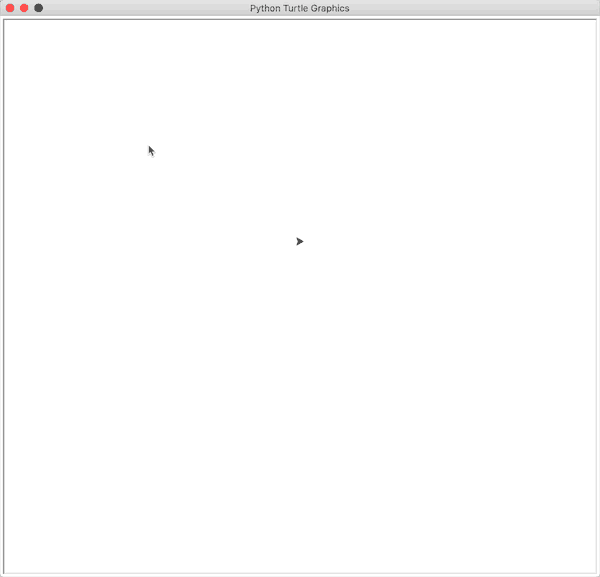
源码:
# -*- coding:utf-8 -*-
import turtle
import math
def shield():
'''
该函数的作用是画一个美国队长的盾牌
'''
# 设置画布背景
turtle.bgcolor('#FFFFFF')
# 设置画笔速度
turtle.speed(10)
# 依次填充同心圆
fill_circle('#FF0000', 230)
fill_circle('#FFFFFF', 178)
fill_circle('#FF0000', 129)
fill_circle('#0000FF', 75)
# 完成五角星
draw_five('#FFFFFF', 75)
# 以下代码,将画好的图案按指定格式保存到当前文件目录
# windows 可以使用.jpg格式,或.ps,MAC使用eps格式,或.ps
ts = turtle.getscreen()
ts.getcanvas().postscript(file="shield.eps")
# 启动事件循环,必须是乌龟图形程序中的最后一个语句
# 如果没有这个语句,代码运行完成后,窗口直接消失。
turtle.done()
def draw_circle(radium):
'''
该函数的作用是画一个圆线
:param radium:半径
'''
# 画笔定位到圆点
turtle.home()
# 提笔
turtle.penup()
# 向前移动指定的半径
turtle.forward(radium)
# 落笔
turtle.pendown()
# 偏转角度
turtle.setheading(90)
# 画一个指定半径的圆
turtle.circle(radium)
# 提笔
turtle.penup()
def fill_circle(color, r1):
'''
该函数的作用是,画一个圆环,有指定的填充色和半径
:param color:颜色
:param r1:半径
'''
# 设置画笔颜色
turtle.pencolor(color)
# 设置填充颜色
turtle.fillcolor(color)
# 开始填充
turtle.begin_fill()
# 画圆线
draw_circle(r1)
# 结束填充
turtle.end_fill()
# 画并填充五角星
def draw_five(color, radium):
'''
该函数的作用是画一个五角星
:param color:颜色
:para radium:
'''
# 画笔定位到圆点
turtle.home()
# 提笔
turtle.penup()
# 偏转90度
turtle.setheading(90)
# 向前移动90个像素
turtle.forward(radium)
# 偏转288度
turtle.setheading(288)
# 落笔
turtle.pendown()
# radians()将角度转换为弧度
long_side = (math.sin(math.radians(36))*radium)/math.sin(math.radians(126))
# 设置画笔颜色
turtle.pencolor(color)
# 设置填充颜色
turtle.fillcolor(color)
# 开始填充
turtle.begin_fill()
for i in range(10):
turtle.forward(long_side)
if i % 2 == 0:
turtle.left(72)
else:
turtle.right(144)
# 结束填充
turtle.end_fill()
# 提笔
turtle.penup()
# 运行主函数
shield()
效果图:
源码:
import turtle
t=turtle.Turtle()
turtle.Turtle().screen.delay(0)
tleft=turtle.Turtle()
#第一部分
t.penup()
t.goto(0,0)
t.pendown()
t.left(20)
t.forward(110)
t.left(25)
t.forward(40)
t.left(100)
t.circle(180,20)
t.right(120)
t.forward(250)
t.left(165)
t.forward(250)
t.right(100)
t.forward(35)
t.left(70)
t.forward(45)
t.left(70)
t.forward(120)
t.left(70)
t.forward(80)
t.left(80)
t.forward(80)
t.left(68)
t.forward(120)
t.left(180)
t.forward(78)
t.right(68)
t.forward(60)
t.right(75)
t.forward(60)
t.right(110)
t.forward(15)
t.left(38)
t.forward(65)
t.right(73)#五边形的直边
t.forward(35)
t.right(70)
t.forward(65)
t.right(68)
t.forward(50)
t.right(80)
t.forward(50)
t.penup()
t.goto(-65,68)
t.pendown()
t.right(7)
t.forward(350)
t.right(165)
t.forward(330)
t.penup()
t.goto(64,65)
t.pendown()
t.left(75)
t.forward(350)
t.left(165)
t.forward(330)
t.penup()
t.goto(300,500)
#第二部分
tleft.left(180)
tleft.right(20)
tleft.forward(110)
tleft.right(25)
tleft.forward(40)
tleft.right(100)
tleft.circle(-180,20)
tleft.left(120)
tleft.forward(250)
tleft.right(165)
tleft.forward(250)
tleft.left(100)
tleft.forward(35)
tleft.penup()
tleft.goto(0,0)
tleft.pendown()
tleft.left(20)
tleft.penup()
tleft.forward(18)
tleft.pendown()
tleft.forward(50)#额头竖线
tleft.penup()
tleft.forward(110)#消除竖线
tleft.pendown()
tleft.left(90)
tleft.forward(30)
tleft.right(90)
tleft.forward(60)
tleft.right(90)
tleft.forward(60)
tleft.right(90)
tleft.forward(60)
tleft.right(90)
tleft.forward(40)
tleft.penup()
tleft.forward(30)
tleft.pendown()
tleft.left(90)
tleft.forward(30)
tleft.right(180)
tleft.forward(100)
tleft.right(90)
tleft.forward(80)
tleft.right(90)
tleft.forward(100)
tleft.penup()
tleft.goto(150,70)
tleft.pendown()
tleft.left(100)
tleft.forward(40)
tleft.right(80)
tleft.circle(-333,40)
tleft.right(160)
tleft.forward(230)
#右半部分
tleft.left(100)
tleft.forward(40)
tleft.left(80)
tleft.forward(20)
tleft.left(100)
tleft.forward(30)
tleft.right(100)
tleft.forward(20)
tleft.right(80)
tleft.forward(30)
tleft.left(80)
tleft.forward(20)
tleft.left(100)
tleft.forward(30)
tleft.right(100)
tleft.forward(20)
tleft.right(80)
tleft.forward(30)
tleft.left(80)
tleft.forward(20)
tleft.left(100)
tleft.forward(30)
tleft.right(100)
tleft.forward(20)
tleft.right(80)
tleft.forward(30)
tleft.left(80)
tleft.forward(20)
tleft.left(100)
tleft.forward(30)
tleft.right(100)
tleft.forward(20)
tleft.right(80)
tleft.forward(30)
tleft.left(80)
tleft.forward(20)
tleft.left(100)
tleft.forward(30)
tleft.right(100)
tleft.forward(20)
tleft.right(80)
tleft.forward(30)
tleft.left(80)
tleft.forward(20)
tleft.left(100)
tleft.forward(30)
tleft.right(100)
tleft.forward(20)
tleft.right(80)
tleft.forward(30)
#右下部分
tleft.left(70)
tleft.forward(30)
tleft.right(110)
tleft.forward(40)
tleft.right(60)
tleft.forward(100)
tleft.right(30)
tleft.circle(200,20)
tleft.left(10)
tleft.forward(80)
#右下部分goto
tleft.penup()
tleft.goto(145,-198)
tleft.pendown()
tleft.left(90)
tleft.forward(30)
tleft.right(30)
tleft.forward(40)
tleft.right(150)
tleft.forward(30)
tleft.backward(30)
tleft.left(90)
tleft.forward(100)
tleft.right(90)
tleft.forward(30)
tleft.backward(30)
tleft.left(90)
tleft.right(30)
tleft.circle(200,20)
tleft.left(10)
tleft.forward(50)
#第三部分脸
t2=turtle.Turtle()
t2.penup()
t2.goto(0,-80)
#尖角
t2.circle(150,extent=90)
t2.pendown()
t2.circle(150,extent=30)
t2.penup()
t2.circle(150,extent=18)
t2.pendown()
t2.circle(150,extent=27)
t2.penup()
t2.circle(150,extent=30)
t2.pendown()
t2.circle(150,extent=27)
t2.penup()
t2.circle(150,extent=18)
t2.pendown()
t2.circle(150,extent=30)
t2.right(100)
t2.forward(40)
#左脸夹
t2.left(80)
t2.circle(333,40)
t2.left(160)
t2.forward(230)
#左半部分
t2.right(100)
t2.forward(40)
t2.right(80)
t2.forward(20)
t2.right(100)
t2.forward(30)
t2.left(100)
t2.forward(20)
t2.left(80)
t2.forward(30)
t2.right(80)
t2.forward(20)
t2.right(100)
t2.forward(30)
t2.left(100)
t2.forward(20)
t2.left(80)
t2.forward(30)
t2.right(80)
t2.forward(20)
t2.right(100)
t2.forward(30)
t2.left(100)
t2.forward(20)
t2.left(80)
t2.forward(30)
t2.right(80)
t2.forward(20)
t2.right(100)
t2.forward(30)
t2.left(100)
t2.forward(20)
t2.left(80)
t2.forward(30)
t2.right(80)
t2.forward(20)
t2.right(100)
t2.forward(30)
t2.left(100)
t2.forward(20)
t2.left(80)
t2.forward(30)
t2.right(80)
t2.forward(20)
t2.right(100)
t2.forward(30)
t2.left(100)
t2.forward(20)
t2.left(80)
t2.forward(30)
t2.right(70)
t2.forward(30)
t2.left(110)
t2.forward(40)
t2.left(60)
t2.forward(100)
t2.left(30)
t2.circle(-200,20)
t2.right(10)
t2.forward(80)
t2.penup()
t2.goto(-145,-198)#左脸颊
t2.pendown()
t2.right(90)
t2.forward(30)
t2.left(30)
t2.forward(40)
t2.left(150)
t2.forward(30)
t2.right(180)
t2.forward(30)
t2.left(90)
t2.forward(100)
t2.left(90)
t2.forward(30)
t2.left(180)
t2.forward(30)
t2.left(120)
t2.circle(-200,20)
t2.right(10)
t2.forward(50)
#左眼
t2.right(135)
t2.forward(70)
t2.left(50)
t2.forward(40)
t2.left(20)
t2.forward(20)
t2.penup()
t2.goto(-100,28)
t2.pendown()
t2.right(70)
t2.forward(65)
t2.left(50)
t2.forward(40)
t2.left(40)
t2.forward(20)
#左眼带
t2.penup()
t2.goto(-105,-10)
t2.pendown()
t2.right(100)
t2.circle(120,extent=20)
t2.circle(60,extent=80)
t2.penup()
t2.goto(-105,-13)
t2.pendown()
t2.right(100)
t2.circle(120,extent=20)
t2.circle(60,extent=80)
t2.penup()
t2.goto(-70,-40)
t2.pendown()
t2.left(10)
t2.forward(30)
t2.penup()
t2.goto(-10,-40)
t2.pendown()
t2.left(35)
t2.forward(30)
t2.penup()
t2.goto(-80,30)
t2.pendown()
t2.right(130)
t2.forward(47)
t2.left(50)
t2.forward(35)
t2.penup()
t2.goto(-60,-45)
t2.pendown()
t2.right(98)
t2.forward(60)
t2.left(20)
t2.forward(80)
t2.left(70)
t2.forward(10)
t2.left(90)
t2.forward(50)
t2.right(60)
t2.forward(30)
t2.right(60)
t2.forward(30)
t2.right(60)
t2.forward(50)
t2.left(90)
t2.forward(10)
t2.left(75)
t2.forward(80)
t2.left(15)
t2.forward(60)
t2.penup()
t2.goto(-80,-140)
t2.pendown()
t2.right(150)
t2.circle(85,extent=45)
t2.left(15)
t2.forward(70)
t2.left(15)
t2.circle(55,extent=55)
t2.penup()
t2.goto(0,-175)
t2.pendown()
t2.left(18)
t2.forward(170)
#右眼
tleft.left(135)
tleft.forward(70)
tleft.right(50)
tleft.forward(40)
tleft.right(20)
tleft.forward(20)
tleft.penup()
tleft.goto(100,28)
tleft.pendown()
tleft.left(70)
tleft.forward(65)
tleft.right(50)
tleft.forward(40)
tleft.right(40)
tleft.forward(20)
#右眼带
tleft.penup()
tleft.goto(105,-10)
tleft.pendown()
tleft.left(100)
tleft.circle(-120,extent=20)
tleft.circle(-60,extent=80)
tleft.penup()
tleft.goto(105,-13)
tleft.pendown()
tleft.left(100)
tleft.circle(-120,extent=20)
tleft.circle(-60,extent=80)
#右眼睛
tleft.penup()
tleft.goto(70,-40)
tleft.pendown()
tleft.right(10)
tleft.forward(30)
tleft.penup()
tleft.goto(10,-40)
tleft.pendown()
tleft.right(35)
tleft.forward(30)
tleft.penup()
tleft.goto(80,30)
tleft.pendown()
tleft.left(130)
tleft.forward(47)
tleft.right(50)
tleft.forward(35)
#鼻子
tleft.penup()
tleft.goto(0,-70)
tleft.pendown()
tleft.left(30)
tleft.forward(20)
tleft.left(72)
tleft.forward(10)
tleft.left(108)
tleft.forward(20)
tleft.right(42)
tleft.forward(20)
tleft.left(108)
tleft.forward(10)
tleft.left(72)
tleft.forward(20)
tleft.penup()
tleft.goto(0,-90)
tleft.pendown()
tleft.left(42)
tleft.forward(20)
tleft.left(72)
tleft.forward(10)
tleft.left(108)
tleft.forward(20)
tleft.right(42)
tleft.forward(20)
tleft.left(108)
tleft.forward(10)
tleft.left(72)
tleft.forward(20)
tleft.penup()
tleft.goto(200,500)
turtle.done()
效果图:
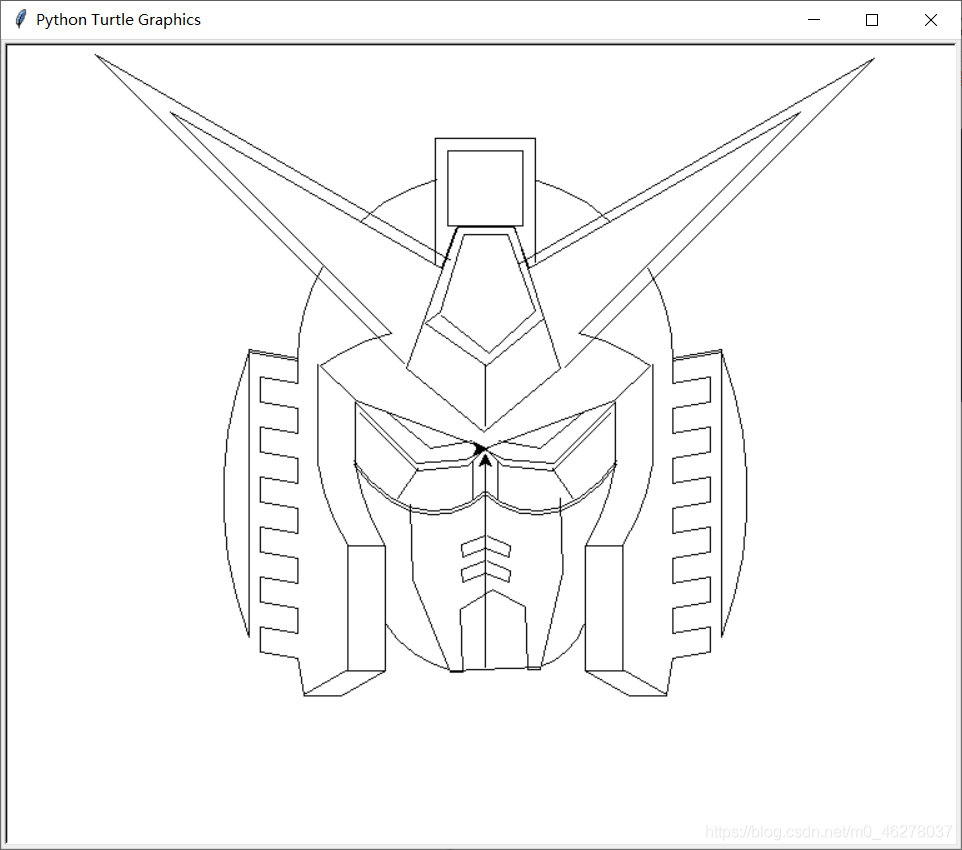
到此这篇关于基于Python-turtle库绘制路飞的草帽骷髅旗、美国队长的盾牌、高达的文章就介绍到这了,更多相关Python-turtle库美国队长的盾牌内容请搜索脚本之家以前的文章或继续浏览下面的相关文章希望大家以后多多支持脚本之家!
您可能感兴趣的文章:- python绘图模块之利用turtle画图
- 详解Python绘图Turtle库
- Python内置模块turtle绘图详解
- python 简单的绘图工具turtle使用详解
- Python趣味挑战之turtle库绘画飘落的银杏树
- 教你利用Python+Turtle绘制简易版爱心表白
- Python使用Turtle模块绘制国旗的方法示例
- python中turtle库的简单使用教程
- Python turtle库的画笔控制说明
- python基于turtle绘制几何图形