目录
- 一、最简单的切换功能
- 二、实现动态切屏功能
- 三、随机效果实现
- 四、效果展现
- 五、第二个版本
- (一)修改了核心代码
- (二)完整代码
- (三)另一种效果
- 六、小结
一、最简单的切换功能
(一)源码
import sys, pygame
import os
import random
pygame.init() # 初始化pygame类
screen = pygame.display.set_mode((600, 600)) # 设置窗口大小
pygame.display.set_caption('美丽的屏保') # 设置窗口标题
tick = pygame.time.Clock()
fps = 10 # 设置刷新率,数字越大刷新率越高
fcclock = pygame.time.Clock()
bglist = []
flag = 0
runimage = None
nextimage = None
def init_image():
path = './image/'
files = []
dirs = os.listdir(path)
for diretion in dirs:
files.append(path + diretion)
for file in files:
picture = pygame.transform.scale(pygame.image.load(file), (600, 600))
dSurface = picture
# dSurface = pygame.image.load(file).convert()
bglist.append(dSurface)
def reset():
global flag,runimage,nextimage
flag = 0
if nextimage is None:
nextimage = random.choice(bglist)
if runimage is None:
runimage = random.choice(bglist)
else:
runimage = nextimage
nextimage = random.choice(bglist)
def run():
global flag,runimage
reset()
while True:
for event in pygame.event.get():
if event.type == pygame.QUIT or event.type == pygame.K_F1:
pygame.quit()
sys.exit()
if event.type == pygame.KEYDOWN:
if event.key == pygame.K_ESCAPE:
pygame.quit()
sys.exit()
if event.key == pygame.K_SPACE:
reset()
if event.type == pygame.MOUSEBUTTONDOWN:
reset()
screen.fill((255, 255, 255)) # 设置背景为白色
screen.blit(nextimage, (0, 0))
screen.blit(runimage, (0, 0))
fcclock.tick(fps)
pygame.display.flip() # 刷新窗口
# time.sleep(10)
if __name__ == '__main__':
init_image()
run()
(二)效果
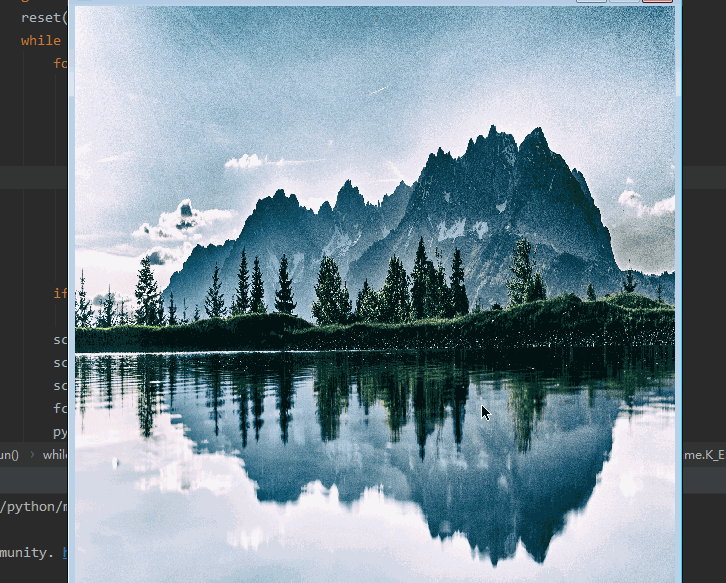
(三)解析
实际就是使用了runimage和nextimage保存两个图片,然后先黏贴nextimage,再黏贴runimage,让runimage显示在最前端。
并通过监听鼠标和键盘操作,每点击一次,切换一次页面。
并调用reset函数
def reset():
global flag,runimage,nextimage
flag = 0
if nextimage is None:
nextimage = random.choice(bglist)
if runimage is None:
runimage = random.choice(bglist)
else:
runimage = nextimage
nextimage = random.choice(bglist)
二、实现动态切屏功能
(一)向左切换
import sys, pygame
import os
import random
pygame.init() # 初始化pygame类
WIDTH = 600
HEIGHT = 600
screen = pygame.display.set_mode((WIDTH, HEIGHT)) # 设置窗口大小
pygame.display.set_caption('美丽的屏保') # 设置窗口标题
tick = pygame.time.Clock()
fps = 60 # 设置刷新率,数字越大刷新率越高
fcclock = pygame.time.Clock()
bglist = []
flag = 0
runimage = None
nextimage = None
flag = False # FALSE没有切屏 TRUE 切屏
flag2 = False
i = 0
j = 0
step = 10
def init_image():
path = './image/'
files = []
dirs = os.listdir(path)
for diretion in dirs:
files.append(path + diretion)
for file in files:
picture = pygame.transform.scale(pygame.image.load(file), (WIDTH, HEIGHT))
dSurface = picture
# dSurface = pygame.image.load(file).convert()
bglist.append(dSurface)
def reset():
global flag,runimage,nextimage,flag2,i,j
flag = False # FALSE没有切屏 TRUE 切屏
flag2 = False
i = 0
j = 0
if nextimage is None:
nextimage = random.choice(bglist)
if runimage is None:
runimage = random.choice(bglist)
else:
runimage = nextimage
nextimage = random.choice(bglist)
def run():
global flag,runimage,flag2,nextimage,i,j
reset()
while True:
for event in pygame.event.get():
if event.type == pygame.QUIT or event.type == pygame.K_F1:
pygame.quit()
sys.exit()
if event.type == pygame.KEYDOWN:
if event.key == pygame.K_ESCAPE:
pygame.quit()
sys.exit()
if event.key == pygame.K_SPACE:
if flag is False:# FALSE没有切屏 TRUE 切屏
flag = True
flag2 = False
# if event.type == pygame.MOUSEBUTTONDOWN:
# reset()
screen.fill((255, 255, 255)) # 设置背景为白色
if flag:
screen.blit(nextimage, (0, 0))
screen.blit(runimage, (i, j))
i -= step
if i = -WIDTH:
flag2 = True
else:
screen.blit(nextimage, (0, 0))
screen.blit(runimage, (0, 0))
if flag2:
reset()
fcclock.tick(fps)
pygame.display.flip() # 刷新窗口
# time.sleep(10)
if __name__ == '__main__':
init_image()
run()
(二)向左切换效果
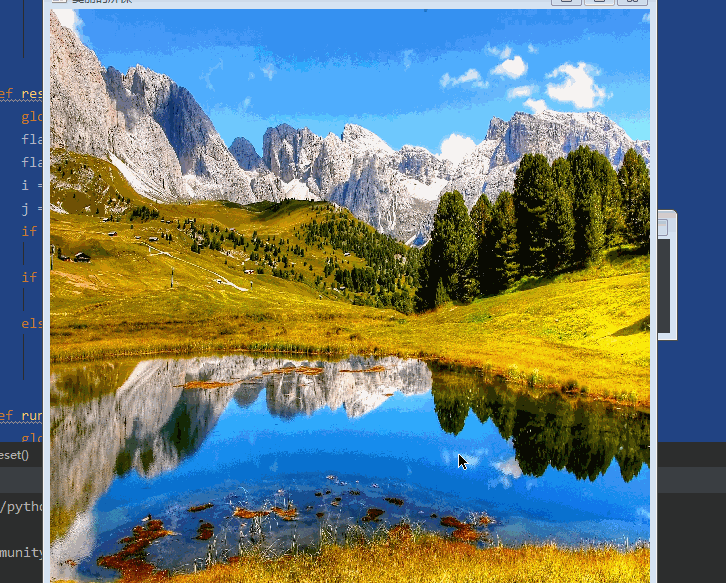
三、随机效果实现
实现上下左右效果
import sys, pygame
import os
import random
pygame.init() # 初始化pygame类
WIDTH = 600
HEIGHT = 600
screen = pygame.display.set_mode((WIDTH, HEIGHT)) # 设置窗口大小
pygame.display.set_caption('美丽的屏保') # 设置窗口标题
tick = pygame.time.Clock()
fps = 60 # 设置刷新率,数字越大刷新率越高
fcclock = pygame.time.Clock()
bglist = []
flag = 0
runimage = None
nextimage = None
flag = False # FALSE没有切屏 TRUE 切屏
flag2 = False
i = 0
j = 0
step = 10
choose = 0
def init_image():
path = './image/'
files = []
dirs = os.listdir(path)
for diretion in dirs:
files.append(path + diretion)
for file in files:
picture = pygame.transform.scale(pygame.image.load(file), (WIDTH, HEIGHT))
dSurface = picture
# dSurface = pygame.image.load(file).convert()
bglist.append(dSurface)
def reset():
global flag,runimage,nextimage,flag2,i,j,choose
flag = False # FALSE没有切屏 TRUE 切屏
flag2 = False
i = 0
j = 0
choose = random.randint(0,3)
if nextimage is None:
nextimage = random.choice(bglist)
if runimage is None:
runimage = random.choice(bglist)
else:
runimage = nextimage
nextimage = random.choice(bglist)
def run():
global flag,runimage,flag2,nextimage,i,j,choose
reset()
while True:
for event in pygame.event.get():
if event.type == pygame.QUIT or event.type == pygame.K_F1:
pygame.quit()
sys.exit()
if event.type == pygame.KEYDOWN:
if event.key == pygame.K_ESCAPE:
pygame.quit()
sys.exit()
if event.key == pygame.K_SPACE:
if flag is False:# FALSE没有切屏 TRUE 切屏
flag = True
flag2 = False
screen.fill((255, 255, 255)) # 设置背景为白色
if flag:
screen.blit(nextimage, (0,0))
print(i+WIDTH,j+HEIGHT)
screen.blit(runimage, (i, j))
if choose==0:
i -= step
if i = -WIDTH:
flag2 = True
elif choose==1:
i += step
if i >= WIDTH:
flag2 = True
elif choose==2:
j -= step
if j = -HEIGHT:
flag2 = True
elif choose==3:
j += step
if j >= HEIGHT:
flag2 = True
else:
screen.blit(nextimage, (0, 0))
screen.blit(runimage, (0, 0))
if flag2:
reset()
# print(choose)
fcclock.tick(fps)
pygame.display.flip() # 刷新窗口
# time.sleep(10)
if __name__ == '__main__':
init_image()
run()
四、效果展现
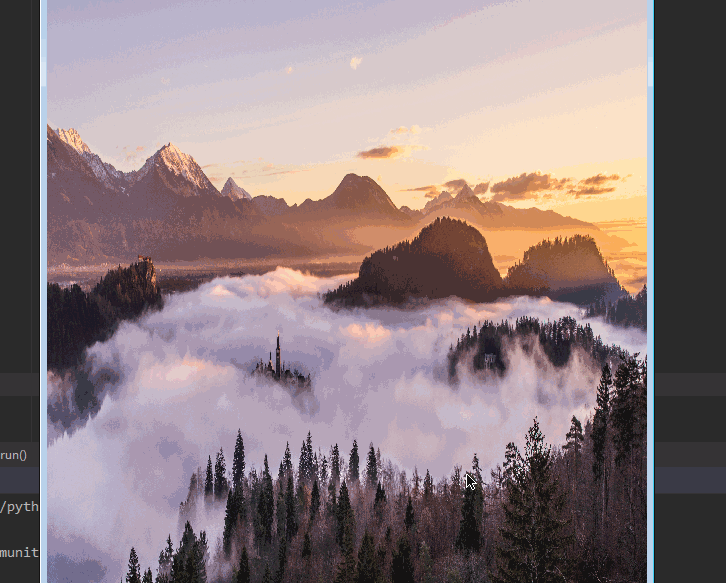
五、第二个版本
(一)修改了核心代码
if flag:
if choose==0:
i -= step
screen.blit(nextimage, (i+WIDTH, 0))
if i = -WIDTH:
flag2 = True
elif choose==1:
screen.blit(nextimage, (i-WIDTH, 0))
i += step
if i >= WIDTH:
flag2 = True
elif choose==2:
screen.blit(nextimage, (0, j+HEIGHT))
j -= step
if j = -HEIGHT:
flag2 = True
elif choose==3:
screen.blit(nextimage, (0, j-HEIGHT))
j += step
if j >= HEIGHT:
flag2 = True
screen.blit(runimage, (i, j))
else:
screen.blit(nextimage, (0, 0))
screen.blit(runimage, (0, 0))
(二)完整代码
import sys, pygame
import os
import random
pygame.init() # 初始化pygame类
WIDTH = 600
HEIGHT = 600
screen = pygame.display.set_mode((WIDTH, HEIGHT)) # 设置窗口大小
pygame.display.set_caption('美丽的屏保') # 设置窗口标题
tick = pygame.time.Clock()
fps = 60 # 设置刷新率,数字越大刷新率越高
fcclock = pygame.time.Clock()
bglist = []
flag = 0
runimage = None
nextimage = None
flag = False # FALSE没有切屏 TRUE 切屏
flag2 = False
i = 0
j = 0
step = 10
choose = 0
def init_image():
path = './image/'
files = []
dirs = os.listdir(path)
for diretion in dirs:
files.append(path + diretion)
for file in files:
picture = pygame.transform.scale(pygame.image.load(file), (WIDTH, HEIGHT))
dSurface = picture
# dSurface = pygame.image.load(file).convert()
bglist.append(dSurface)
def reset():
global flag,runimage,nextimage,flag2,i,j,choose
flag = False # FALSE没有切屏 TRUE 切屏
flag2 = False
i = 0
j = 0
choose = random.randint(0,3)
if nextimage is None:
nextimage = random.choice(bglist)
if runimage is None:
runimage = random.choice(bglist)
else:
runimage = nextimage
nextimage = random.choice(bglist)
def run():
global flag,runimage,flag2,nextimage,i,j,choose
reset()
while True:
for event in pygame.event.get():
if event.type == pygame.QUIT or event.type == pygame.K_F1:
pygame.quit()
sys.exit()
if event.type == pygame.KEYDOWN:
if event.key == pygame.K_ESCAPE:
pygame.quit()
sys.exit()
if event.key == pygame.K_SPACE:
if flag is False:# FALSE没有切屏 TRUE 切屏
flag = True
flag2 = False
screen.fill((255, 255, 255)) # 设置背景为白色
if flag:
if choose==0:
i -= step
screen.blit(nextimage, (i+WIDTH, 0))
if i = -WIDTH:
flag2 = True
elif choose==1:
screen.blit(nextimage, (i-WIDTH, 0))
i += step
if i >= WIDTH:
flag2 = True
elif choose==2:
screen.blit(nextimage, (0, j+HEIGHT))
j -= step
if j = -HEIGHT:
flag2 = True
elif choose==3:
screen.blit(nextimage, (0, j-HEIGHT))
j += step
if j >= HEIGHT:
flag2 = True
screen.blit(runimage, (i, j))
else:
screen.blit(nextimage, (0, 0))
screen.blit(runimage, (0, 0))
if flag2:
reset()
# print(choose)
fcclock.tick(fps)
pygame.display.flip() # 刷新窗口
# time.sleep(10)
if __name__ == '__main__':
init_image()
run()
(三)另一种效果
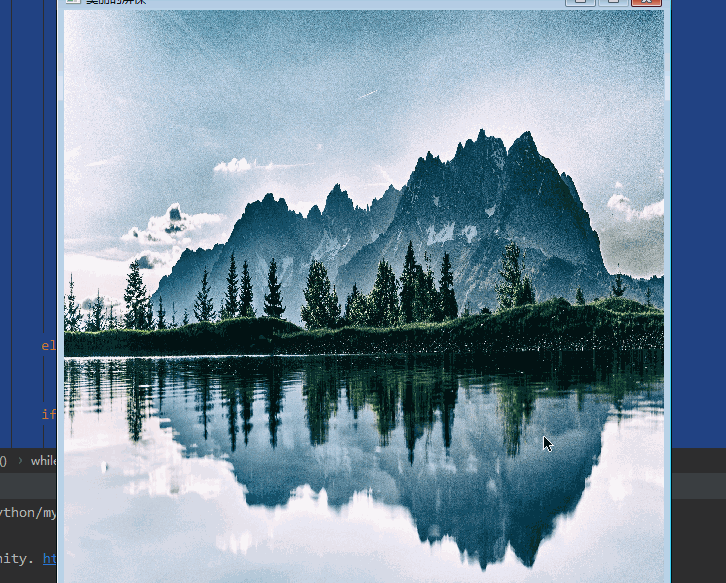
六、小结
Ok,V1和V2版本,两个版本,任君选择,比较简单,大家将就着看看啊。后面会有修订和更多有趣的案例,欢迎关注,感谢支持!
以上就是pygame实现类似office的页面切换功能的详细内容,更多关于pygame页面切换的资料请关注脚本之家其它相关文章!
您可能感兴趣的文章:- pygame多种方式实现屏保操作(自动切换、鼠标切换、键盘切换)