目录
- 一、初始化主界面
- 二、第一种进度条
- 三、第二种进度条
- 四、第三种进度条
- 五、第四种进度条
- 六、综合案例
一、初始化主界面
import pygame
pygame.init()
screen = pygame.display.set_mode((500,300))
pygame.display.set_caption("好看的进度条显示V1.0")
clock = pygame.time.Clock()
while True:
for event in pygame.event.get():
if event.type == pygame.QUIT or event.type == pygame.K_F1:
pygame.quit()
sys.exit()
screen.fill((255,255,255))
clock.tick(30)
pygame.display.flip()
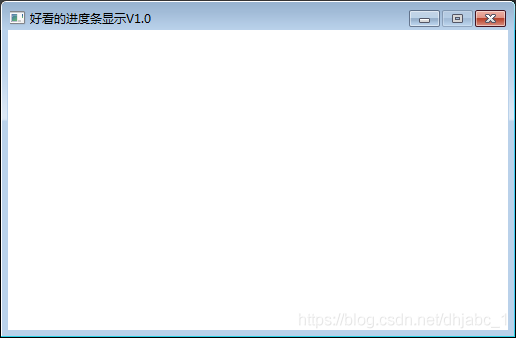
二、第一种进度条
(一)核心代码
pygame.draw.rect(screen,(192,192,192),(5,100,490,20))
pygame.draw.rect(screen,(0,0,255),(5,100,step,20))
(二)设置步长,并循环递增
(三)完整代码
import pygame,sys
pygame.init()
screen = pygame.display.set_mode((500,300))
pygame.display.set_caption("好看的进度条显示V1.0")
clock = pygame.time.Clock()
step = 0
while True:
for event in pygame.event.get():
if event.type == pygame.QUIT or event.type == pygame.K_F1:
pygame.quit()
sys.exit()
screen.fill((255,255,255))
# screen.fill((0,0,0))
pygame.draw.rect(screen,(192,192,192),(5,100,490,20))
pygame.draw.rect(screen,(0,0,255),(5,100,step % 490,20))
step += 1
clock.tick(60)
pygame.display.flip()
(四)运行效果
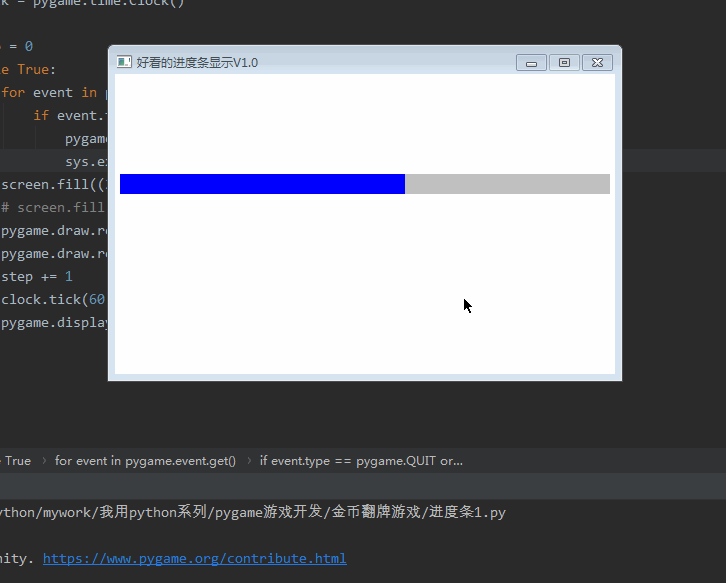
三、第二种进度条
(一)核心代码
pygame.draw.rect(screen,(192,192,192),(5,100,490,20))
pygame.draw.rect(screen,(0,0,255),(5,100,step % 490,20))
font1 = pygame.font.Font(r'C:\Windows\Fonts\simsun.ttc', 16)
text1 = font1.render('%s %%' % str(int((step % 490)/490*100)), True, (255,0,0))
screen.blit(text1, (245, 100))
(二)完整代码
import pygame,sys
pygame.init()
screen = pygame.display.set_mode((500,300))
pygame.display.set_caption("好看的进度条显示V1.0")
clock = pygame.time.Clock()
step = 0
while True:
for event in pygame.event.get():
if event.type == pygame.QUIT or event.type == pygame.K_F1:
pygame.quit()
sys.exit()
screen.fill((255,255,255))
# screen.fill((0,0,0))
pygame.draw.rect(screen,(192,192,192),(5,100,490,20))
pygame.draw.rect(screen,(0,0,255),(5,100,step % 490,20))
font1 = pygame.font.Font(r'C:\Windows\Fonts\simsun.ttc', 16)
text1 = font1.render('%s %%' % str(int((step % 490)/490*100)), True, (255,0,0))
screen.blit(text1, (245, 100))
step += 1
clock.tick(60)
pygame.display.flip()
(三)运行结果

四、第三种进度条
(一)核心代码
pygame.draw.rect(screen,(192,192,192),(5,100,length+10,20))
pygame.draw.rect(screen,(0,0,255),(5,100,step % length,20))
pygame.draw.circle(screen,(0,0,255),(step % length,110),10)
font1 = pygame.font.Font(r'C:\Windows\Fonts\simsun.ttc', 16)
text1 = font1.render('%s %%' % str(int((step % length)/length*100)), True, (255,0,0))
screen.blit(text1, (245, 100))
(二)完整代码
import pygame,sys
pygame.init()
screen = pygame.display.set_mode((500,300))
pygame.display.set_caption("好看的进度条显示V1.0")
clock = pygame.time.Clock()
step = 0
length = 480
while True:
for event in pygame.event.get():
if event.type == pygame.QUIT or event.type == pygame.K_F1:
pygame.quit()
sys.exit()
screen.fill((255,255,255))
# screen.fill((0,0,0))
pygame.draw.rect(screen,(192,192,192),(5,100,length+10,20))
pygame.draw.rect(screen,(0,0,255),(5,100,step % length,20))
pygame.draw.circle(screen,(0,0,255),(step % length,110),10)
font1 = pygame.font.Font(r'C:\Windows\Fonts\simsun.ttc', 16)
text1 = font1.render('%s %%' % str(int((step % length)/length*100)), True, (255,0,0))
screen.blit(text1, (245, 100))
step += 1
clock.tick(60)
pygame.display.flip()
(三)运行效果
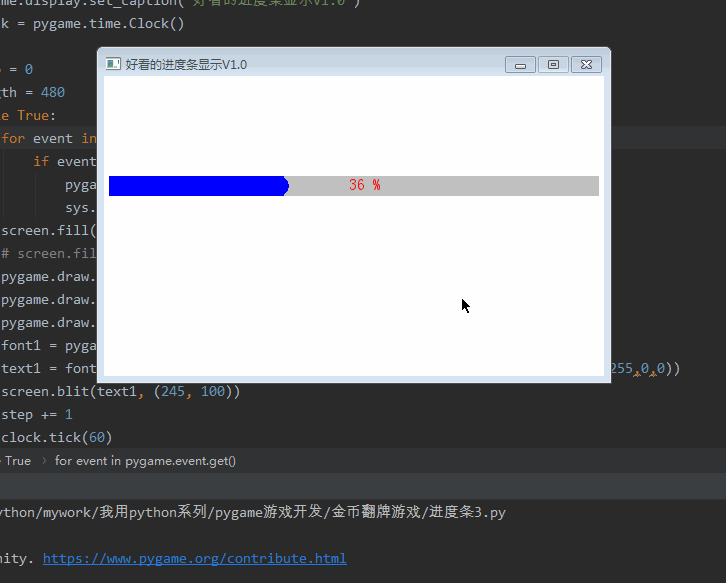
五、第四种进度条
(一)加载图片资源
picture = pygame.transform.scale(pygame.image.load('score/5.png'), (20, 20))
(二)画进度条
pygame.draw.rect(screen,(192,192,192),(5,100,length+10,20))
pygame.draw.rect(screen,(251,174,63),(5,100,step % length,20))
(三)画图片资源
screen.blit(picture,(step%length,100))
(四)画文字
font1 = pygame.font.Font(r'C:\Windows\Fonts\simsun.ttc', 16)
text1 = font1.render('%s %%' % str(int((step % length)/length*100)), True, (255,0,0))
screen.blit(text1, (245, 100))
(五)完整代码
import pygame,sys
pygame.init()
screen = pygame.display.set_mode((500,300))
pygame.display.set_caption("好看的进度条显示V1.0")
clock = pygame.time.Clock()
picture = pygame.transform.scale(pygame.image.load('score/5.png'), (20, 20))
step = 0
length = 480
while True:
for event in pygame.event.get():
if event.type == pygame.QUIT or event.type == pygame.K_F1:
pygame.quit()
sys.exit()
screen.fill((255,255,255))
# screen.fill((0,0,0))
pygame.draw.rect(screen,(192,192,192),(5,100,length+10,20))
pygame.draw.rect(screen,(251,174,63),(5,100,step % length,20))
screen.blit(picture,(step%length,100))
font1 = pygame.font.Font(r'C:\Windows\Fonts\simsun.ttc', 16)
text1 = font1.render('%s %%' % str(int((step % length)/length*100)), True, (255,0,0))
screen.blit(text1, (245, 100))
step += 1
clock.tick(60)
pygame.display.flip()
(六)运行效果
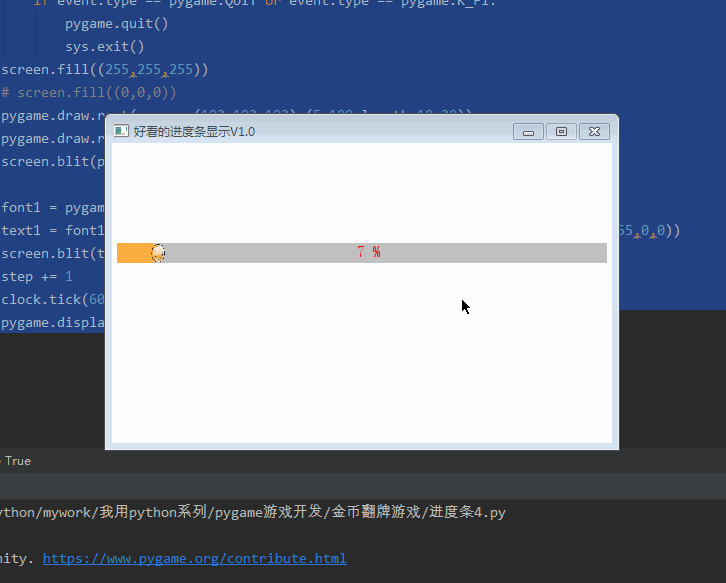
六、综合案例
(一)完整代码
import pygame,sys
pygame.init()
screen = pygame.display.set_mode((500,300))
pygame.display.set_caption("好看的进度条显示V1.0")
clock = pygame.time.Clock()
picture = pygame.transform.scale(pygame.image.load('score/5.png'), (20, 20))
step = 0
length = 480
while True:
for event in pygame.event.get():
if event.type == pygame.QUIT or event.type == pygame.K_F1:
pygame.quit()
sys.exit()
screen.fill((255,255,255))
# screen.fill((0,0,0))
# 第一种
pygame.draw.rect(screen,(192,192,192),(5,100,490,20))
pygame.draw.rect(screen,(0,0,255),(5,100,step % 490,20))
# 第二种
pygame.draw.rect(screen,(192,192,192),(5,150,490,20))
pygame.draw.rect(screen,(0,0,255),(5,150,step % 490,20))
font1 = pygame.font.Font(r'C:\Windows\Fonts\simsun.ttc', 16)
text1 = font1.render('%s %%' % str(int((step % 490)/490*100)), True, (255,0,0))
screen.blit(text1, (245, 150))
# 第三种
pygame.draw.rect(screen,(192,192,192),(5,200,length+10,20))
pygame.draw.rect(screen,(0,0,255),(5,200,step % length,20))
pygame.draw.circle(screen,(0,0,255),(step % length,210),10)
font1 = pygame.font.Font(r'C:\Windows\Fonts\simsun.ttc', 16)
text1 = font1.render('%s %%' % str(int((step % length)/length*100)), True, (255,0,0))
screen.blit(text1, (245, 200))
# 第四种
pygame.draw.rect(screen,(192,192,192),(5,250,length+10,20))
pygame.draw.rect(screen,(251,174,63),(5,250,step % length,20))
screen.blit(picture,(step%length,250))
font1 = pygame.font.Font(r'C:\Windows\Fonts\simsun.ttc', 16)
text1 = font1.render('%s %%' % str(int((step % length)/length*100)), True, (255,0,0))
screen.blit(text1, (245, 250))
step += 1
clock.tick(60)
pygame.display.flip()
(二)运行效果
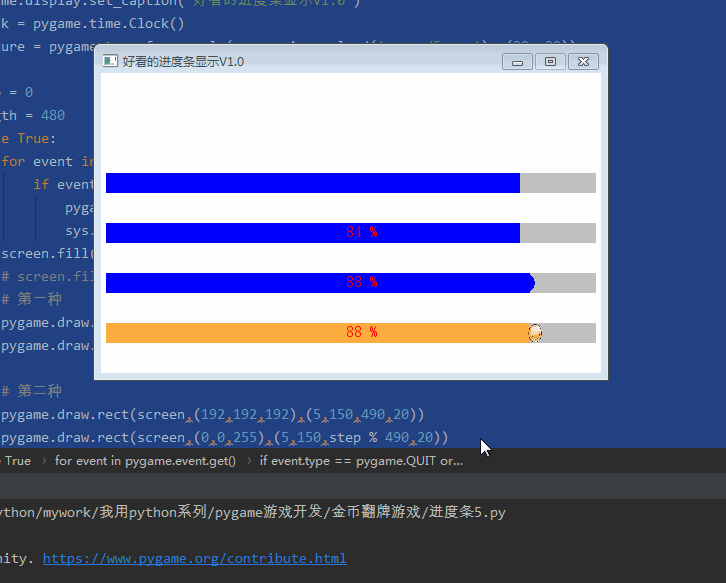
OK,写完,本博文纯属科普贴,技术含量不高,入门级别,大家喜欢就好。
而且里面代码相对比较简单,也没有考虑优化,大家在实操过程中可以优化完善,并反馈给我一起进步。
到此这篇关于Python趣味挑战之教你用pygame画进度条的文章就介绍到这了,更多相关pygame画进度条内容请搜索脚本之家以前的文章或继续浏览下面的相关文章希望大家以后多多支持脚本之家!
您可能感兴趣的文章:- Python趣味挑战之pygame实现无敌好看的百叶窗动态效果
- Python趣味挑战之用pygame实现简单的金币旋转效果
- Python3+Pygame实现射击游戏完整代码
- python 基于pygame实现俄罗斯方块
- python pygame 愤怒的小鸟游戏示例代码
- Python3.9.0 a1安装pygame出错解决全过程(小结)
- python之pygame模块实现飞机大战完整代码
- Python使用Pygame绘制时钟
- Python3.8安装Pygame教程步骤详解
- python pygame入门教程